python单进程
ping
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| import subprocess
def ping(host): result = subprocess.run( 'ping -c2 %s &> /dev/null' % host,shell=True ) if result.returncode == 0: print('%s:up'% host) else: print('%s:down'% host)
if __name__ == '__main__': ips = ['192.168.0.%s' %i for i in range(1,255)] for ip in ips: ping(ip)
|
这个会ping得很慢,因为要等待前一个进程得到结果才能运行下一个命令
多进程
ping2
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| import subprocess import os
def ping(host): result = subprocess.run( 'ping -c2 %s &> /dev/null' % host,shell=True ) if result.returncode == 0: print('%s:up'% host) else: print('%s:down'% host)
if __name__ == '__main__': ips = ['192.168.0.%s' %i for i in range(1,255)] for ip in ips: ret_val = os.fork() if not ret_val: ping(ip) exit()
|
这个就会很快,因为用了多进程的概念(注意:是多进程不是多线程)
Windows是没有多进程的概念的,只有多线程;Linux有多进程和多线程的概念,两者要注意分开!!!!
产生僵死进程
frozen_process
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| import os import time
print('starting') ret_val = os.fork()
if ret_val: print('in parent') time.sleep(30) print('parent done') else: print('in child') time.sleep(15) print('child done')
|
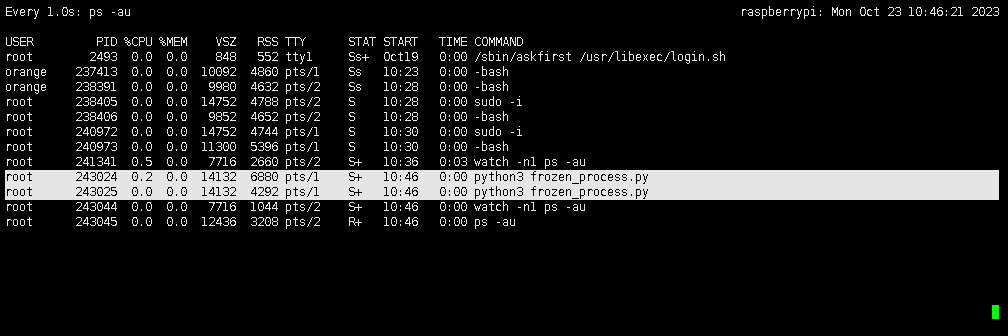
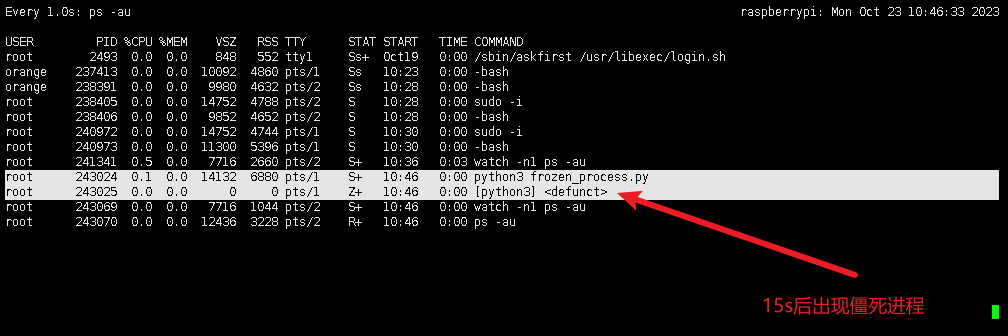
线程挂起与不挂起_os.waitpid()
1 2
| os.waitpid(-1,0) os.waitpid(-1,1)
|
挂起父进程
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| import os import time
print('starting') ret_val = os.fork()
if ret_val: print('in parent') result = os.waitpid(-1,0) print(result) time.sleep(3) print('parent done') else: print('in child') time.sleep(5) print('child done')
|
父进程print('in parent')
,然后运行到os.waitpid(-1,0)
父进程挂起,子进程干活print('in child')
,睡觉5秒后print('child done')
,父进程开始杀死子进程,并且打印print(result)
,睡觉3秒后print('parent done')
不挂起父进程
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| import os import time
print('starting') ret_val = os.fork()
if ret_val: print('in parent') result = os.waitpid(-1,1) print(result) time.sleep(10) print('parent done') else: print('in child') time.sleep(5) print('child done')
|